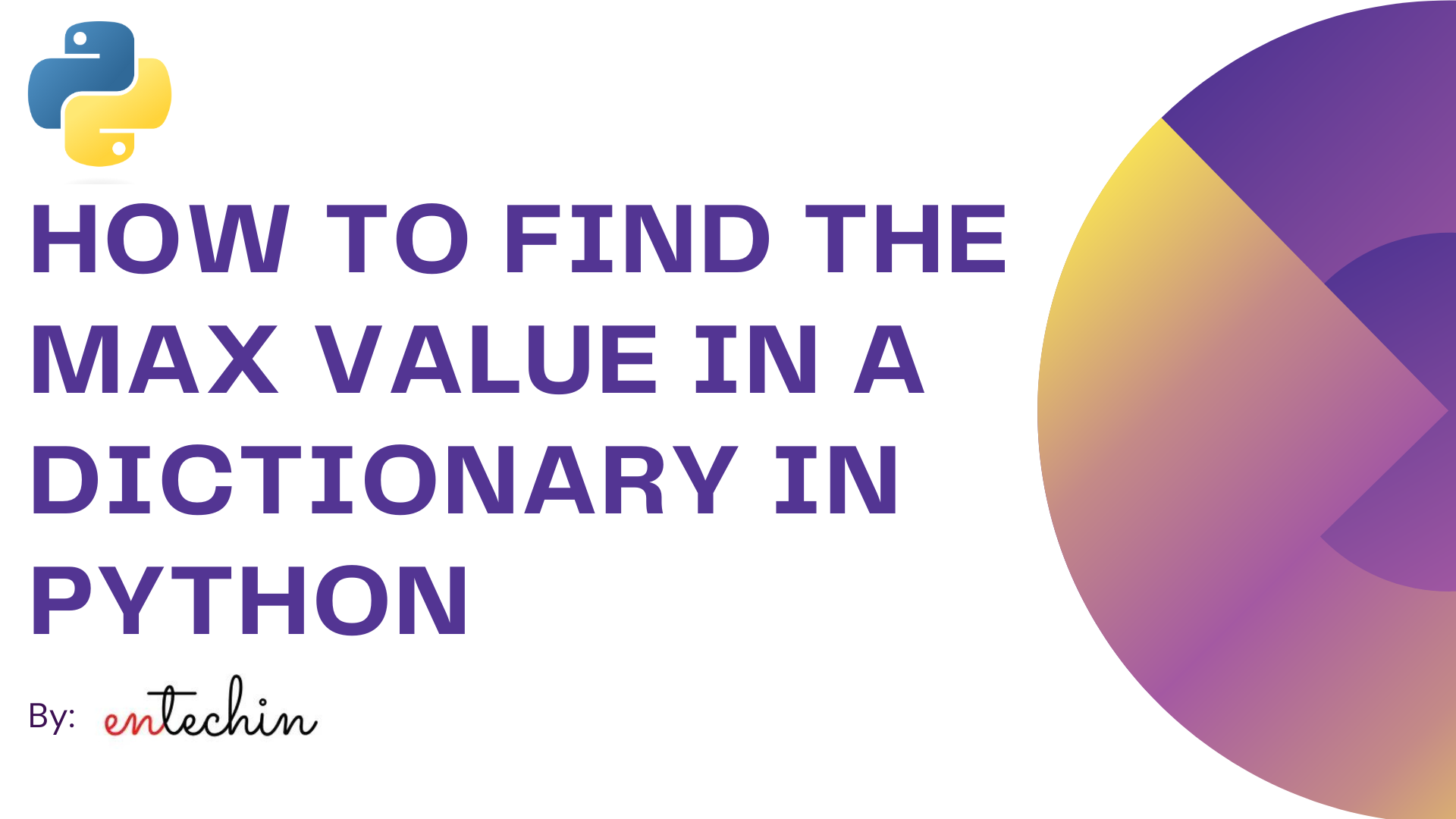
In Python, dictionaries are data structures that can efficiently store data as key-value pairs. They allow for fast and efficient retrieval of values based on their associated keys. Unlike sequences like as lists and tuples, which use numerical indexes to access data, dictionaries use keys to access data. Python dictionaries provide the flexibility to easily add, modify, and update entries. In this article, we will explore different methods to find the max value in a Python dictionary.
Core Concepts:
- Dictionaries: Unordered collections of key-value pairs. Keys are unique and immutable (cannot be changed after creation), while values can be of any data type (numbers, strings, lists, etc.).
- max() Function: A built-in function that returns the largest element within an iterable (like a list or dictionary) or the highest of two or more arguments provided to it.
Methods for Finding Maximum value in a Dictionary in Python
There are four main methods to find the maximum value in a Python dictionary:
1) Max() with Get() Function: Ideal for custom criteria. This method involves using the max() function with the get() function, particularly useful for custom criteria.
2) Max() and Lambda Function: Combine max()
with a lambda function to find the maximum element based on a custom criterion defined within the lambda function.
3) Using Values() and Keys() Function: This simple approach involves using the values() and keys() functions to find the maximum value in a dictionary.
4) Using Values() Along With List Comprehension: This method utilizes list comprehension to directly access the key associated with the maximum value in a dictionary.
1. Using Max() with get() Function
In Python, dictionaries store data in key-value pairs. The get() function retrieves a value associated with a specific key, while the max() function typically returns the largest value in a list or among arguments. However, when used directly with dictionaries, max() returns the key with the highest value, not the value itself.
To find the actual maximum value within a dictionary, leverage max() with the key argument. This argument lets you define a custom function that calculates a comparison value for each element. max() then returns the key associated with the highest calculated value.
Example: Discover the Top Student with Highest Score:
This approach is perfect for scenarios like finding the student with the highest score in a dictionary of exam results. Here’s a step-by-step guide:
- Create a Dictionary: Define a dictionary named
scores
where keys are student names and values are their scores (e.g.,scores = {'Susan': 92, 'Olivia': 78, ...}
). - Find Top Student: Use
max()
with thekey
argument set toscores.get
. This instructs Python to compare the values returned byget()
for each student. The result will be the key (student name) with the highest score. - Print the Result: Display the name of the top student and their corresponding score.
scores = {'Susan': 92, 'Olivia': 78, 'Samantha': 88, 'Edward': 95, 'George': 83}
top_student = max(scores, key=scores.get)
print(f"The top student is {top_student} with a score of {scores[top_student]}."
Output:
The top student is Edward with a score of 95.
In this example, we created a dictionary named scores, where keys represent student names, and values represent their scores. Then, we used the max() function with the key parameter set to scores.get. The scores.get function is applied to each value in the dictionary to extract the value that will be compared. In this case, it retrieves the score associated with each student’s name. The max() function then identifies the key (student name) with the highest score based on the values returned by scores.get. The result of max() is stored in the variable top_student, which holds the name of the student with the highest score.
2. Using max() and Lambda Function
- Lambda functions are small, anonymous functions used for concise, one-time operations.
- Combine max() with a lambda function to find the maximum element based on a custom criterion defined by the lambda function.
- Provide the lambda function as the
key
argument to max(). This function calculates a value for each element in the iterable, which max() uses to determine the maximum element.
# Define a dictionary
sales = {
'January': 55000,
'February': 62000,
'March': 72000,
'April': 69000,
'May': 78000,
'June': 85000
}
# Use the 'max()' function to find the key (month) with the maximum value (highest sales).
best_sales_month = max(sales, key=lambda month: sales[month])
# Print the result, indicating the month with the highest sales.
print("Best Sales Month:", best_sales_month)
Output:
Best Sales Month: June
In this code, the ‘key’ parameter within the max() function informs the function to perform comparisons based on the dictionary’s values, specifically the sales amounts. Then we used a lambda function as the key function. This lambda function, defined as lambda month: sales[month], operates on an argument ‘month’, which iterates through each month in the dictionary. It extracts the corresponding sales amount using sales[month]. On the other hand, the max() function identifies the month associated with the highest sales by considering the values returned by the lambda function.
Upon executing this code, the output will display the name of the month with the highest sales. In this example, it would print ‘June’ as the result.
3. Using Values() and Keys() Function
This is the simplest approach to finding the key with the maximum value in a dictionary. Python dictionaries store data in key-value pairs. But how do you identify the key with the highest corresponding value? This is where values() and keys() functions come in handy.
- values(): Returns a view of all values in the dictionary.
- keys(): Returns a view of all keys in the dictionary.
Here’s an example to demonstrate how you can use these two functions i.e. values() and keys() to find the maximum value in a dictionary. In this example, we created a dictionary “genre_frequencies” which represents the frequency of different book genres in a library’s collection. The code then identifies and displays the most popular book genre and the number of books in that genre in the library’s collection.
# Suppose you have a dictionary that represents the frequency of book genres in a library's collection.
genre_frequencies = {
'Mystery': 45,
'Science Fiction': 31,
'Romance': 22,
'Fantasy': 58,
'Biography': 14,
'History': 36,
'Self-Help': 19,
'Cooking': 27,
'Thriller': 36,
'Science': 42
}
# Get all the keys (genres) and values (frequencies) using keys() and values() methods.
genres = list(genre_frequencies.keys())
frequencies = list(genre_frequencies.values())
# Find the index of the maximum frequency in the list of frequencies.
max_frequency_index = frequencies.index(max(frequencies))
# Use the index to find the corresponding genre.
most_popular_genre = list(genres)[max_frequency_index]
# Print the result using an f-string.
print(f"In the library's collection, the most popular book genre is '{most_popular_genre}' with {max(frequencies)} books.")
Output:
In the library's collection, the most popular book genre is 'Fantasy' with 58 books.
In this example, we first used the keys() and values() methods to obtain separate lists of genres and frequencies. Then, we find the index of the maximum frequency in the list of frequencies using the index() method. Finally, we used this index to retrieve the corresponding genre and displayed the result.
4. Using Values() along with List Comprehension
List comprehension provides a more efficient and expressive solution that allows you to directly access key with maximum value. Here’s an example demonstrating how to use list comprehension to find the key associated with the maximum value in a dictionary:
# Suppose you have a dictionary representing the scores of different players.
player_scores = {
'Player1': 95,
'Player2': 88,
'Player3': 92,
'Player4': 88,
'Player5': 90
}
# Find the maximum score in the dictionary.
max_score = max(player_scores.values())
# Use list comprehension to get a list of players with the maximum score.
top_players = [player for player, score in player_scores.items() if score == max_score]
# Print the result using an f-string.
print(f"The top players with the highest score ({max_score}) are: {', '.join(top_players)}")
Output:
The top players with the highest score (95) are: Player1
In this example, we have a dictionary called player_scores, where the keys are player names, and the values are their scores. We first find the maximum score using the max() function.
Then, we use list comprehension to create a list of player names (top_players) where the score matches the maximum score. Finally, we print out the top players with the highest score, and if there are multiple players with the same top score, they will all be listed.
Extracting Maximum Values from Dictionaries of Lists in Python
In data analysis, dictionaries are a cornerstone for storing and organizing complex data. They excel at representing hierarchical relationships, where a single key can be associated with various values. However, these values can extend beyond simple data types and encompass entire lists. This capability empowers us to represent rich datasets like sales figures for individual salespeople over time.
Consider a scenario where sales_data is a dictionary containing sales figures for various salespeople:
sales_data = {
'John': [3500, 4200, 3800, 4500], # Sales figures for John
'Sarah': [4200, 3900, 4100, 4300], # Sales figures for Sarah
'Michael': [3800, 4000, 3950, 4100] # Sales figures for Michael
}
In this scenario, the sales_data dictionary contains lists of sales figures for different salespeople over a specific time period. Each key (salesperson’s name) corresponds to a list of sales figures, where each element in the list represents sales for a particular time period (e.g., monthly sales).
Identifying the Top Salesperson
To determine the salesperson with the highest overall sales, we can leverage Python’s built-in functionalities. Here’s the code achieving this:
# Find the maximum sales figure across all salespeople
max_sales = max(max(sales_data[person]) for person in sales_data)
print("The maximum sales figure is:", max_sales)
Output:
The maximum sales figure is: 4500
In the above code, we iterate through each person (key) in the sales_data dictionary. For each person, we find the maximum sales figure within their respective list of sales figures using max(sales_data[person]). Finally, we find the maximum of these maximums, which represents the overall maximum sales figure across all sales people.
Unveiling the Top Performer’s Identity:
We can extend our analysis to identify the name of the salesperson who achieved this top performance. Here’s the modified code:
sales_data = {
'John': [3500, 4200, 3800, 4500], # Sales figures for John
'Sarah': [4200, 3900, 4100, 4300], # Sales figures for Sarah
'Michael': [3800, 4000, 3950, 4100] # Sales figures for Michael
}
# Find the maximum sales figure across all salespeople
max_sales = max(max(sales_data[person]) for person in sales_data)
# Find the name of the person with the maximum sales
person_with_max_sales = [person for person in sales_data if max_sales in sales_data[person]][0]
print("The maximum sales figure is:", max_sales)
print("The person with the maximum sales is:", person_with_max_sales)
Output:
The maximum sales figure is: 4500
The person with the maximum sales is: John
In this code, we first find the maximum sales figure (max_sales) across all salespeople, just like before. Then, we use a list comprehension to find the name of the person whose sales figures list contains the max_sales value. This person’s name is stored in the person_with_max_sales variable, and we print it out.
Find the max value in Nested dictionaries
In some scenarios, you may encounter nested dictionaries, and the value you want to compare for finding the maximum resides deep within these nested structures. To tackle this, you’ll need to navigate through multiple layers of key-value pairs within dictionaries within dictionaries. Let’s discuss an example that demonstrates how you can find the key with the maximum value in a nested dictionary.
Imagine you have a dictionary that represents a sports league, and for each team, there’s a dictionary containing the number of wins, losses, and draws. You want to find the team with the highest number of wins.
# Define a dictionary 'sports_league' with team information
sports_league = {
'Team A': {'wins': 12, 'losses': 5, 'draws': 3},
'Team B': {'wins': 9, 'losses': 8, 'draws': 3},
'Team C': {'wins': 15, 'losses': 2, 'draws': 3},
'Team D': {'wins': 10, 'losses': 6, 'draws': 4}
}
# Use the 'max()' function with a custom key to find the team with the most wins
top_team = max(sports_league, key=lambda team: sports_league[team]['wins'])
# Print the name of the top team and their number of wins
print(f"The top team is {top_team} with {sports_league[top_team]['wins']} wins.")
Output:
The top team is Team C with 15 wins.
Consider another example in which you have a dictionary representing a class of students, and each student has multiple exam scores. You want to find the student with the highest average score and print their name. Now, this example is slightly different. In this case, you need to compute the average score of all the nested dictionaries and then find the maximum among them.
# Define a dictionary 'student_scores' with student information
student_scores = {
'Alice': {'scores': [90, 85, 88, 92, 95]},
'Bob': {'scores': [78, 88, 92, 80, 85]},
'Charlie': {'scores': [92, 95, 88, 90, 78]},
'David': {'scores': [85, 88, 92, 90, 78]}
}
# Function to calculate the average score for a student
def calculate_average(scores):
return sum(scores) / len(scores)
# Use a list comprehension to calculate the average score for each student
average_scores = {student: calculate_average(data['scores']) for student, data in student_scores.items()}
# Find the student with the highest average score
top_student = max(average_scores, key=average_scores.get)
# Print the name of the top student and their average score
print(f"The top student is {top_student} with an average score of {average_scores[top_student]}.")
Output:
The top student is Alice with an average score of 90.0.
In this example, we first calculate the average score for each student using a list comprehension. Then, we use the max function with a custom key to find the student with the highest average score. Finally, we print the name of the top student and their average score as the result.
Conclusion
In summary, we’ve explored various methods to find the maximum value within a Python dictionary. While dictionaries are versatile data structures, navigating nested dictionaries or dictionaries with complex structures can sometimes pose challenges when finding the maximum value.
We’ve covered methods such as using the max() function with custom key functions, employing the get() function, using lambda functions, and list comprehensions. You can even use loops for more intricate operations. These methods, although diverse in their approach, allow you to efficiently identify the maximum value within dictionaries of varying complexities. For any questions or queries, contact us.
If you want to learn more about Python programming, visit Python Programming Tutorials.