
In this tutorial, we will see How to convert any binary string to and from ASCII text in Python. ASCII stands for American Standard Code For Information Interchange. It is a standard 8-bit encoding format that assigns numerical values to other characters in a computer such as letters, punctuation marks, etc.
In Python, you can convert a binary string to ASCII text and vice versa using the built-in functions and methods. To convert a binary string to ASCII text, you can use the int
function with base 2 to convert the binary string to an integer, followed by the chr
function to convert the integer to its corresponding ASCII character. For example:
binary_string = '01101000 01100101 01101100 01101100 01101111'
ascii_text = ''.join(chr(int(binary, 2)) for binary in binary_string.split())
print(ascii_text)
Conversely, to convert ASCII text to a binary string, you can use the ord
function to get the ASCII value of each character and then use the format
function to convert the ASCII value to its binary representation. For example:
ascii_text = 'Hello'
binary_string = ' '.join(format(ord(char), '08b') for char in ascii_text)
print(binary_string)
Finding ASCII Value
The ASCII value of ‘K’ is 75. You can check the ASCII value of different characters by executing the code given below. It takes an input character from the user and displays its ASCII value using the ord() function.
# Program to find the ASCII value of the given character
char = str(input("Enter any character: "))
print("The ASCII value of '" + char + "' is", ord(char))
OUTPUT:
Enter any character: a
The ASCII value of 'a' is 97
The ord() function only works for a character. If you want to obtain the ASCII value of all characters in a string then use a for loop to access all elements one by one. Convert them into ascii using ord() function and append the value in another variable.
Computers store data in the form of binary numbers i.e. 1’s and 0’s. Suppose you want to perform some operations or manipulate a string that is stored in the computer memory. For this, you need to convert this binary string to ASCII value to retrieve the original string. This article discusses different ways to convert a binary string into ASCII value using Python language.
To learn more about Python Programming, visit Python Programming Tutorials.
Converting Binary string or text to ASCII
1. Using binascii module
Binascii module aids in the conversion of binary strings to their equivalent ASCII representation. First of all, import the library of binascii and then take a binary string as input from the user. You can also convert a string to binary representation by inserting “b” at the start of an input string. b2a.uu() is a binascii function that converts the binary string to ASCII representation.
import binascii
# Initializing a binary string
Text = b"This is my string"
ASCII = binascii.b2a_uu(Text)
# Getting the ASCII equivalent
print(ASCII)
b"15&AI<R!I<R!M>2!S=')I;F< \n"
2. Using int.to_ byte() function
The first step is to initialize a binary string using the int(binary_input, base) command. Pass the string of 0s and 1s in the first argument and the base of the number system in the second argument. Before moving towards coding, let’s first understand how a binary string composed of bits of 0s and 1s is converted into an ASCII value. You already know eight bits are equal to 1 byte. Suppose you have a binary string as shown below. In order to find the ASCII value, we first grouped 8 bits. A group of 8 bits represents 1 byte which represents 1 character.
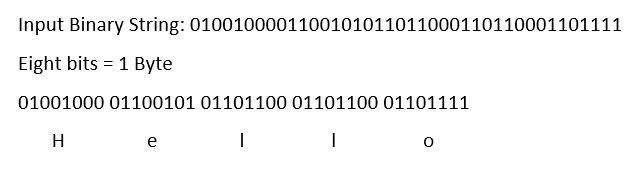
The first step is to find the number of bytes in a binary string which is done using (input_string.bit_length() +7) // 8 command. Here, input_string.bit_length() returns the total number of bits in an input string. After this, convert it into ASCII text using the decoder() function. The complete code is given below.
# Initialize a binary string
input_string=int("0100100001100101011011000110110001101111", 2);
#Obtain the total number of bytes
Total_bytes= (input_string.bit_length() +7) // 8
#Convert these bits to bytes
input_array = input_string.to_bytes(Total_bytes, "big")
#Convert the bytes to an ASCII value and display it on the output screen
ASCII_value=input_array.decode()
print(ASCII_value)
OUTPUT:
Hello
3. Using Int.from_bytes() function (ASCII to Binary Text)
The above two methods were related to the conversion of binary to ASCII. In this method, we will learn to convert ASCII to Binary. For this, convert the string into an array using string.encode() function. Then call the function int.from_byte() to convert the array of bytes into a binary integer which is then passed to the bin() function to obtain a binary string of 0s and 1s.
input_array = "Hello".encode()
binary_array= int.from_bytes(input_array, "big")
output_string = bin(binary_array)
print(output_string)
0b100100001100101011011000110110001101111
This tutorial is all about the conversion of binary from ASCII and vice versa. Similarly, you can also convert other number systems such as decimal numbers, and hexadecimal numbers into binary numbers and vice versa.
If you have any feedback about this article, do let us know. To learn more about Python language, visit this link.