
The colored or RGB images are composed of three color channels which are red, green and blue. Each pixel of an RGB image is composed of varying proportions of red, green, and blue colors which are added together to produce any color in the range of a visible spectrum.
In image processing, working with color images (RGB) can be computationally expensive. To streamline processing, we often convert them to grayscale or black and white formats. This tutorial guides you through these conversions in Python using the popular OpenCV and Pillow libraries.
Grayscale vs. Black and White
- Grayscale Images: These images retain shades of gray, with each pixel value ranging from 0 (black) to 255 (white). They offer a more detailed representation than black and white images.
- Black and White Images: These images contain only two distinct colors: black (0) and white (255). They are suitable for simpler processing tasks.
Python provides different modules for image conversion. Some of the common ways are discussed here. If you want to learn more about Python Programming, visit Python Programming Tutorials.
Convert an image Into Black And White using openCV
A simple binary thresholding technique in OpenCV can be used to convert an image to black and white. We establish a threshold value and each pixel value is compared with this threshold. If the pixel value is less than this threshold, it is set to 0. The pixel value is set to 1 if the pixel value is greater than this threshold. Here, 0 represents black color and 1 represents white color.
To apply thresholding, first of all, we need to convert a colored image to grayscale. Read the image by providing the path of the image in ‘imread(“path of image”)’ command. Then, convert this image to grayscale using cv2.cvtColor(image, color_space_conversion) function. Here, the first parameter specifies the input to be transformed. The opencv read the image in BGR mode which is same as RGB mode. The second parameter i.e., color_space_conversion specifies the color space from which you want to transform and the color pace in which you want to transform. In this case, we are converting BGR mode to grayscale that’s why we have used cv2.COLOR_BGR2GRAY. The next step is the conversion of this grayscale image to black and white image. For this, we’ll apply thresholding as discussed above. The syntax of a binary thresholding function is:
cv2.threshold (image, threshold, max_value, cv2.THRESH_BINARY)
In the above, ‘image’ is the grayscale image that is to be transformed. The second parameter specifies the threshold value with which all pixel values are compared. Then, the third input parameter is set. If the pixel value is greater than the threshold value, it is changed to the value of parameter “max-value”. In binarization, we want the maximum value to be 255 as the pixel value for the black color is 255.
import cv2
from google.colab.patches import cv2_imshow
#read the image
image = cv2.imread('C:/Users/abc/Desktop/penguins.jpg')
#Convert an image from BGR to grayscale mode
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#Convert a grayscale image to black and white using binary thresholding
(thresh, BnW_image) = cv2.threshold(gray_image, 125, 255, cv2.THRESH_BINARY)
#display all the images
cv2_imshow(image)
cv2_imshow(gray_image)
cv2_imshow(BnW_image)
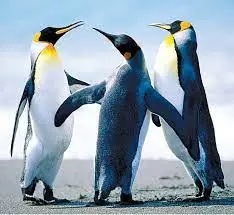
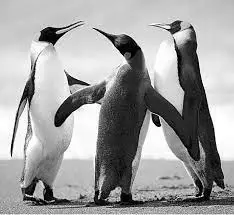

Explanation:
- cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) converts the image from BGR (OpenCV’s default color space) to grayscale.
- cv2.threshold(gray_image, 125, 255, cv2.THRESH_BINARY)
From the output, it can be seen that in the grayscale images, each pixel value lie in a range of 0 to 255 represented different shades of gray. On other hand, black and white images consists of either 0 (white) or 1 (Black) pixels.re
Convert image from White to Black using Pillow library
Python pillow library provides a .convert() function to convert the images into grayscale or the black and white mode. The syntax of this function is:
image.convert(‘mode’)
Here, ‘image’ corresponds to the input image which is to be transformed. If you want to transform an image to grayscale, then insert ‘L’ in the mode. This mode has only one channel whose value lies in a range of 0 to 255. It represents black, white and all the shades of gray. The L mode transforms the image to grayscale mode. Use ‘1’ in mode to transform the image into black and white mode. To study in detail about pillow library or .convert() method, visit this link. Now, lets move towards the code.
from PIL import Image
#read the image from path
image = Image.open('C:/Users/abc/Desktop/penguins.jpg')
#Convert it into the grayscale image
grayscale = image.convert('L')
#Converting the same image to black and white mode
BW= image.convert('1')
#save both the images
grayscale.save("grayscale_image.jpg")
BW.save("BW_image.jpg")
Execute the above code and then open the saved files. The output is shown below.
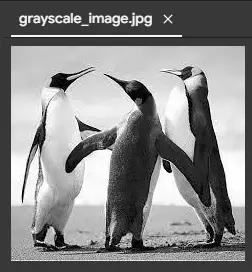
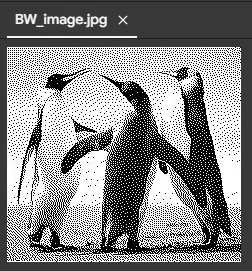
The above code produced the black and white image with dithering which is basically noise. The convert() function has an argument dithering. To disable dithering, it is set to NONE.
from PIL import Image
#read the image
color_image = Image.open('C:/Users/abc/Desktop/penguins.jpg')
#convert the image to black and white mode with dither set to None
bw = color_image.convert('1', dither=Image.NONE)
#save the image with name "BW_image.jpg"
bw.save('BW_image.jpg')
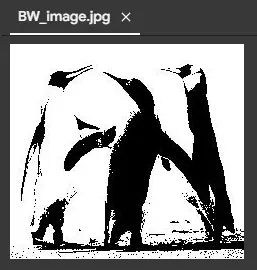
The images are converted to either grayscale or black and white because grayscale images are easier to process as compared to the coloured images. It plays a vital role in image processing applications.
Choosing the Right Method
- If you need precise control over the the black and white conversion using thresholding, OpenCV is a good choice.
- If speed and simplicity are priorities, Pillow’s
convert()
function might be preferable.
If you have any questions, please let us know. Your feedback matters a lot for us.