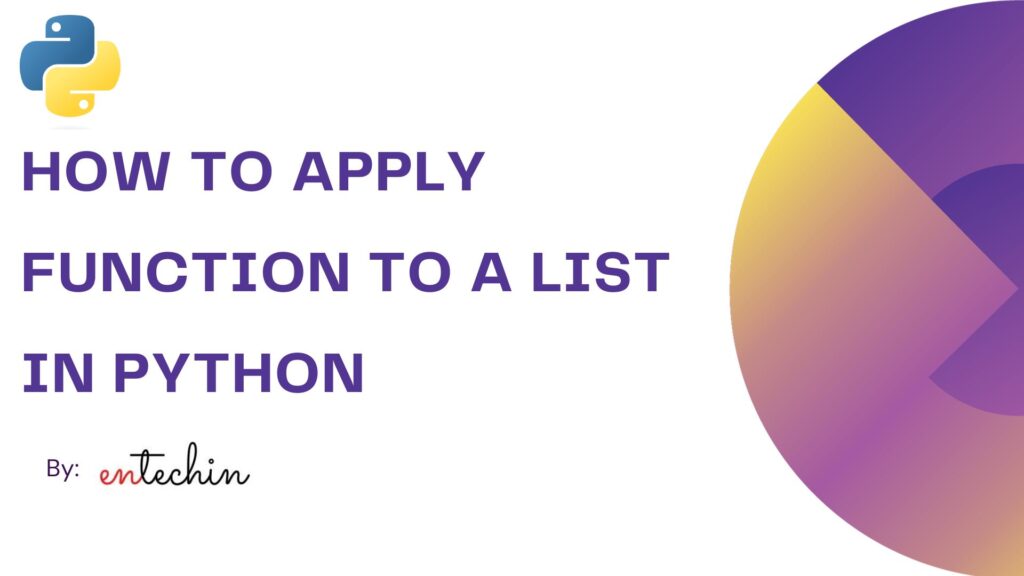
Applying a function to a list is a fundamental concept in programming. It enables us to perform operations on each element of a list effectively. In this tutorial, we will explore different methods to apply a function to a list in Python. Applying a function to each element of a list can be achieved through various approaches. Let’s discuss them in detail.
Methods for Applying a Function to a List in Python
1. Using For loop
One of the simplest ways to apply a function to each element of a list is by using a for loop. Let’s consider an example where you have a list of strings and you want to convert each string to uppercase.
words = ["apple", "banana", "cherry", "date"]
# Create an empty list to store the uppercase strings
uppercase_words = []
# Iterate over each word in the list
for word in words:
# Apply the upper() function to convert the word to uppercase
uppercase_word = word.upper()
# Append the uppercase word to the new list
uppercase_words.append(uppercase_word)
# Print the list of uppercase words
print(uppercase_words)
OUTPUT:
['APPLE', 'BANANA', 'CHERRY', 'DATE']
The output shows the uppercase versions of the words in the words
list.
In this example, we have used a for loop to iterate over each element in the words
list. Inside the loop, we apply the upper()
function to convert each word to uppercase. The uppercase word is then appended to the uppercase_words
list. Finally, we print the list of uppercase words.
The for loop gives you more flexibility in applying complex functions or performing conditional operations on each element of a list. It allows you to customize the function application based on your specific requirements.
2. Using map() Function (Applying to all elements of a list).
The map() function in Python provides an efficient way to apply a function to each element in a list. It takes two arguments: the function to be applied and the iterable (list) on which the function will be applied. The map() function returns a map object, which can be converted to a list using the list() function.
Let’s consider an interesting example to illustrate the usage of the map() function. Suppose you have a list of numbers and you want to calculate the square of each number.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Define a function to calculate the square of a number
def square(x):
return x ** 2
# Apply the square function to each element in the numbers list using the Map() function
squared_numbers = list(map(square, numbers))
# Print the list of squared numbers
print(squared_numbers)
Output:
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
In this example, we define a function called square()
that takes a number x
as input and returns the square of x
. Here, the map() function apply the square()
function to each element in the numbers
list. The result is a map object, which is converted to a list using the list() function. Finally, we print the list of squared numbers.
The map() function offers several other advantages, such as compact and readable code, improved performance for large datasets, and the ability to apply complex functions to each element in a list. It is a powerful tool for data transformation and manipulation in Python.
3. Using list comprehension
Another concise way is to use list comprehension to apply a function to each element in a list. It allows you to combine the iteration and transformation steps into a single line of code.
Suppose you have a list of names and you want to create a new list containing the lengths of these names. Using list comprehension, you can achieve this in just one line of code:
# Create a list of names
names = ['Alice', 'Bob', 'Charlie', 'David']
# Use list comprehension to calculate the length of each name and create a new list
name_lengths = [len(name) for name in names]
# Print the list of name lengths
print(name_lengths)
Output:
[5, 3, 7, 5]
In this code, we start with a list of names. Then, using list comprehension, we iterate over each name in the names
list and calculate the length of each name using the len()
function. The result is a new list called name_lengths
which contains the lengths of the names. Finally, we print the name_lengths
list.
List comprehension simplifies the process of applying a function to a list. You can perform various operations on a list in a compact manner using this method.
4. Using Lambda Function
Lambda functions, also known as anonymous functions, are a convenient way to define small, one-line functions without explicitly using the def
keyword. Combined with the map()
or filter()
function, they provide a powerful tool for applying a function to each element of a list.
Suppose you have a list of temperatures in Celsius and you want to convert them to Fahrenheit. Using a lambda function and the filter()
function, you can easily perform the conversion:
# Define a lambda function to filter even numbers
is_even = lambda x: x % 2 == 0
# Create a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Filter the list based on the condition using map()
result = list(filter(is_even, numbers))
# Print the result
print(result)
OUTPUT:
[2, 4, 6, 8, 10]
Certainly! Here’s another example that demonstrates how to use a lambda function with map()
to filter a list based on a condition:
# Define a lambda function to filter even numbers
is_even = lambda x: x % 2 == 0
# Create a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Filter the list based on the condition using filter()
result = list(filter(is_even, numbers))
# Print the result
print(result)
Output:
csharpCopy code[2, 4, 6, 8, 10]
In this example, we defined a lambda function is_even
that checks if a given number is even by using the modulo operator %
to check if the remainder is zero. By using filter(is_even, numbers)
, we applied the is_even
function as a filter to each element of the numbers
list. The result was converted into a list using the list()
function and stored in the result
variable. The output prints the filtered list, containing only the even numbers.
You can modify the lambda function and the list to apply different conditions and filters to the elements of the list using map()
and lambda functions. This method is particularly useful when you need to perform simple calculations or transformations on the elements of a list.
Conclusion
In conclusion, applying a function to a list in Python provides a powerful way to process and transform data efficiently. By utilizing techniques such as lambda functions, the map()
or filter()
function, for loops, or list comprehensions, you have various options to iterate over each element of a list and apply a specific function to it. These flexible tools enable you to streamline your code, enhance code readability, and achieve desired outcomes in a concise and effective manner. Whether you are working with numerical data, textual information, or any other type of data, applying functions to lists empowers you to handle and manipulate data with ease in Python.
For any queries related to applying a function to a list in Python, Contact Us.